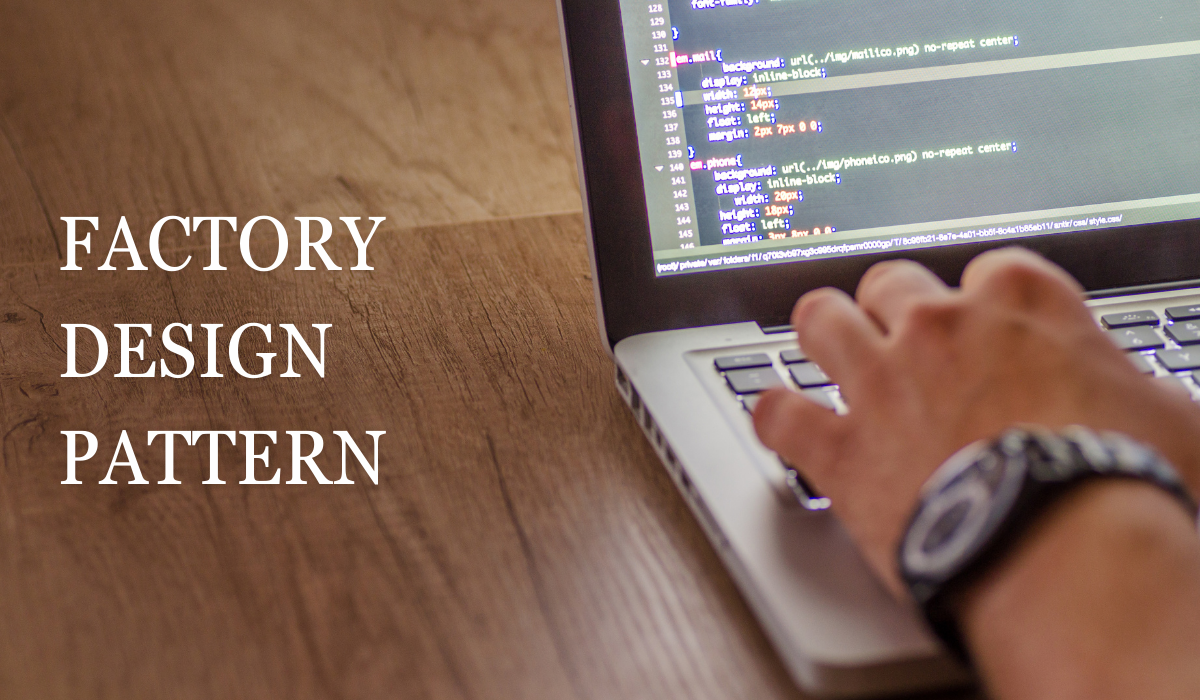
What is the Factory Design Pattern?
The Factory Design Pattern is a creational design pattern that provides an interface for creating objects in a super class, but allows subclasses to alter the type of objects that will be created. In simpler terms, it defines an interface for creating an object but leaves the choice of its type to the subclasses, creating instances of a class without specifying their concrete classes.
Where to Use the Factory Design Pattern?
The Factory Design Pattern is particularly useful in the following scenarios:
- Complex Object Creation: When the process of object creation is complex or involves multiple steps, the Factory pattern helps encapsulate and simplify the object creation process.
- Class Independence: When a system is configured with multiple classes and the client code should be independent of how its objects are created, composed, and represented.
Where Not to Use Factory?
- Simple Object Creation: If the object creation process is straightforward and does not involve multiple steps, using a factory might introduce unnecessary complexity.
- Tight Coupling: If the client code is already aware of the concrete classes it needs to instantiate, introducing a factory may add unnecessary abstraction and increase coupling.
How to Decide Whether to Use a Factory or Not?
Consider using a Factory when:
- There is a need to delegate the responsibility of instantiating objects to subclasses.
- The object creation process is complex or involves multiple steps.
- The client code should be independent of the concrete classes it creates.
Code Example
function Developer(name) {
this.name = name;
this.type = 'Developer';
}
function Tester(name) {
this.name = name;
this.type = 'Tester';
}
function EmployeeFactory() {
this.create = (name, type) => {
switch (type) {
case 1:
return new Developer(name);
case 2:
return new Tester(name);
}
};
}
const employeeFactory = new EmployeeFactory();
const employee = [];
function sayHi() {
console.log('Hi, I am ' + this.name + ', I am a ' + this.type);
}
employee.push(employeeFactory.create('Divesh', 1));
employee.push(employeeFactory.create('Ridam', 2));
employee.push(employeeFactory.create('Sujit', 1));
employee.map((emp) => {
sayHi.call(emp);
});
Code Explanation
- We have two classes,
Developer
andTester
, representing different types of employees. - The
EmployeeFactory
encapsulates the object creation logic. It has acreate
method that takes a name and a type, and based on the type, it creates an instance of the corresponding class. - The client code uses the factory to create instances of employees without directly dealing with their concrete classes.
- The
sayHi
function is a method added to the prototype of both classes, providing a common interface for interacting with the created objects. - Finally, we create instances of employees using the factory and call the
sayHi
method on each of them.
By using the Factory Design Pattern, we achieve a separation of concerns, making the client code unaware of the specific employee class it’s working with, thus promoting flexibility and maintainability in our code.