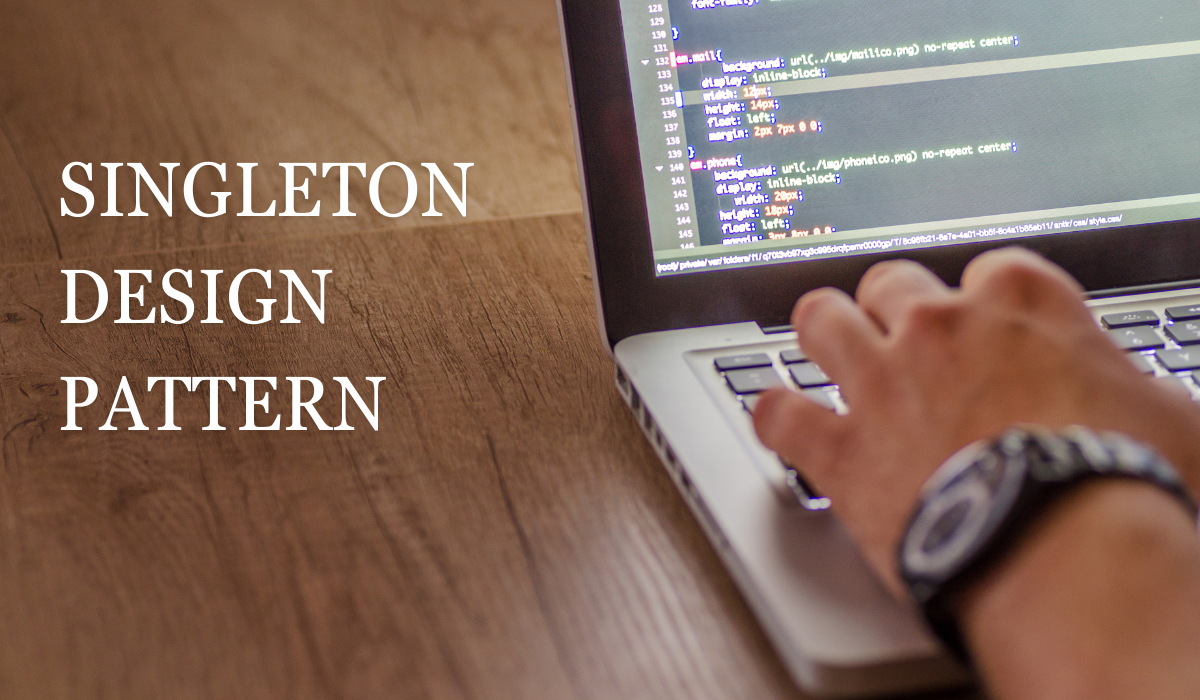
What is the Singleton Design Pattern?
The Singleton Design Pattern is a creational design pattern that ensures a class has only one instance and provides a global point of access to that instance. It is useful when exactly one object is needed to coordinate actions across the system, such as a single point of control for a resource.
Where to Use the Singleton Design Pattern?
Use the Singleton pattern when:
- There must be exactly one instance of a class, and it must be accessible from a well-known access point.
- Controlling access to resources, such as a database connection pool or a logging service, where multiple instances could cause issues.
Where Not to Use Singleton?
Avoid using Singleton when:
- Overuse may lead to a global state that is difficult to control.
- The global state maintained by a Singleton can make it challenging to test the code.
How to Decide Whether to Use a Singleton or Not?
Consider using a Singleton when:
- There is a need for a single point of control for a resource or service.
- A single instance of a class is required for coordinating actions across the system.
Code Example
function Process(state) {
this.state = state;
}
const Singleton = (function () {
function ProcessManager() {
this.numOfProcess = 0;
}
var pManager;
function createProcessManager() {
pManager = new ProcessManager();
return pManager;
}
return {
getProcessManager: () => {
if (!pManager) {
pManager = createProcessManager();
}
return pManager;
},
};
})();
const processManager1 = Singleton.getProcessManager();
const processManager2 = Singleton.getProcessManager();
console.log(processManager1 === processManager2);
Code Explanation
- We have a
Process
class representing some process with a state. - The Singleton pattern is implemented using an immediately-invoked function expression (IIFE) to create a closure, encapsulating the creation of the single instance of
ProcessManager
. - The
ProcessManager
class is designed to have only one instance, and thecreateProcessManager
function is used to instantiate it. - The Singleton object exposes a
getProcessManager
method, ensuring that there is only one instance ofProcessManager
. If it doesn’t exist, it is created; otherwise, the existing instance is returned. - Two instances of
processManager
are created using the Singleton, and the comparisonprocessManager1 === processManager2
confirms that they are the same instance, demonstrating the Singleton pattern’s single instance behavior.